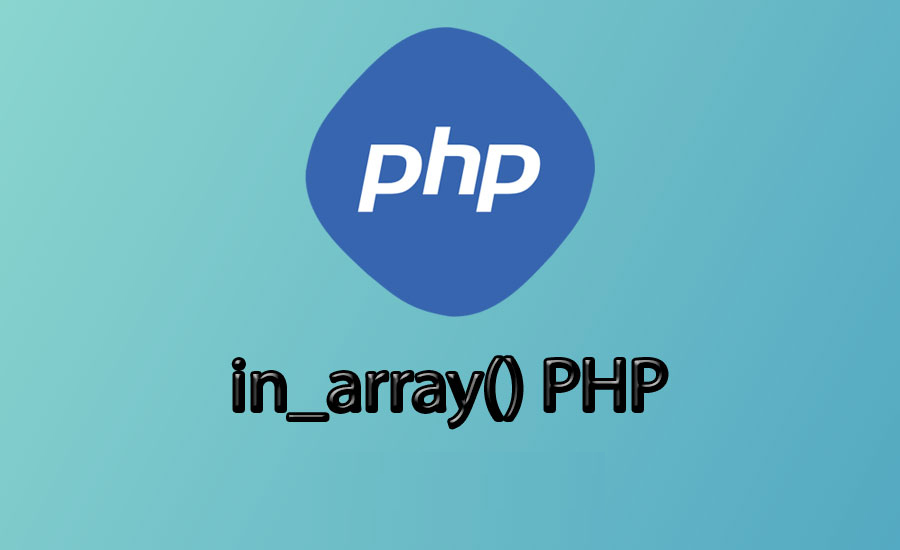
The Guide to Using the in_array() Function in PHP
1. Introduction
In PHP programming, verifying the existence of an element within an array is a common requirement. The in_array()
function serves as a powerful tool to conveniently and efficiently accomplish this task. Below is an in-depth article with numerous illustrative examples to help you understand how to utilize this function.
2. Syntax of the in_array()
Function in PHP
in_array($value_to_check, $array, $strict);
Example
$fruits = array("apple", "banana", "orange");
if (in_array("banana", $fruits)) {
echo "Exists in the array";
} else {
echo "Does not exist";
}
3. Differentiating in_array()
and array_search()
in PHP
While both functions are used to search for a value within an array, they have significant differences. The following example illustrates this:
$fruits = array("apple", "banana", "orange");
$index = array_search("banana", $fruits);
if ($index !== false) {
echo "Index of 'banana' is: $index";
} else {
echo "'banana' does not exist in the array";
}
4. Common Issues When Using the in_array()
Function
Be mindful of data types to avoid unexpected results. For example:
$numbers = array(1, 2, 3, "4", 5);
if (in_array("4", $numbers)) {
echo "'4' exists in the array";
} else {
echo "'4' does not exist in the array";
}
5. Distinction Between in_array()
, array_search()
, and array_keys()
To comprehend the differences better, consider the following example:
$colors = array("red", "green", "blue", "green");
$key_array = array_keys($colors, "green");
print_r($key_array);
6. Using the in_array()
Function in Real-world Applications
Apply in_array()
to check the existence of a product in a shopping cart:
$cart = array("item1", "item2", "item3");
if (in_array("item2", $cart)) {
echo "The product is already in the shopping cart";
} else {
echo "Add the product to the shopping cart";
}
7. Advanced Examples
Check the existence of a string in an array of strings:
$words = array("apple", "banana", "orange");
$search = "nana";
if (in_array($search, $words)) {
echo "$search exists in the array";
} else {
echo "$search does not exist in the array";
}
8. Tips and Tricks
- Use
$strict = true
to compare data types. - Check the array before using
in_array()
to avoid errors.
9. Reference Documentation and Useful Sources
This article not only enhances your understanding of the in_array()
function but also provides practical examples for flexible application in your PHP programming endeavors.
Keywords:
- PHP array push
- PHP in_array
- In_array Laravel
- Array_search
- array exist php
- In_array
- array in php
- Array_intersect